IMDB non-commercial datasets schema
IMDb provides a subset of their data in tab-separated format for personal and non-commercial use. You can find more information, including legal, at IMDb Non-Commercial Datasets. These are some notes on the schema.
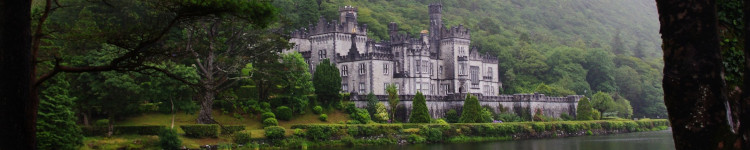
Create an EC2 key pair with ssh-keygen and deploy with AWS CDK
You can create a key pair that will be used to SSH into EC2 instances with:
ssh-keygen -m PEM -b 4096 -f ~/.ssh/my-key-pair -t rsa
This will create two files in ~/.ssh/
, one with the private key named my-key-pair
and one with the public key named
my-key-pair.pub
. Parameters:
-m
: The key format.-t
: The key type. At the time of this writing on 2023-06-13, EC2 supports RSA and ED25519 keys.-b
: The key bits.-f
: The file name in which the key will be stored. Two files will be generated, one for the private and one for the public key.
You can use the public key to create an EC2 key pair and associate it with your EC2 instances. Here's a minimal CDK code snippet for this:
import { Stack, StackProps } from 'aws-cdk-lib';
import * as ec2 from 'aws-cdk-lib/aws-ec2';
import { Construct } from 'constructs';
export class MyStack extends Stack {
constructor(scope: Construct, id: string, props?: StackProps) {
super(scope, id, props);
const cfnKeyPair = new ec2.CfnKeyPair(this, 'MyCfnKeyPair', {
keyName: 'keyName',
publicKeyMaterial: [
'ssh-rsa AAAAB3NzaC1yc2EAAAADAQABAAACAQCtS/sY98Yk6RqJXrWQIqMrRoesRKTI0s6xRUlSPJzx7G8kbWKEH1YS+kE0xFOfdbo/MpXpU',
'yFf9vTIKS5HEG5ZKhFnLpbh3fBBfFmkFNazJcxpyu4yGQyy8SEhavM8xMl1NCpIhBmg8fccn78FwHVjrwBDaXlLkCkHkQf5AM+Fgx2lEOuSNz',
'4NmIvDBAEzJi8gixgKlZM5wnyEOHXyUQ04Xs+vS6RHLxmBQ90ncmMga9FhflqfmSC8r/1uMVQYgW+8/pXOGvbMRmdy9zxxnIz6EBcNtAyWhGO',
'sWB743fdXpCpbIqtiMXImkpjnItU15ar9ij+vkgB5nKBBqFbIvlQ0IKYZ5VJxZMFlpRNZAVyEDedcDWSvc8As5APYau/UgdEv73ingEZpqZR5',
'VcpKQfP4F3psgHtIO+cyPvKss0Q0vKPMwmpl7z5RRcbKxWGXizsQ+B9kvVs3HzK8gu4qaDW1RbEyWkdIzOkV+ovnhqzbn9o6078hkdIU62wix',
'k7fI9ugiOEFLoTiiAUo2H/nQ+Z06I+rxrOgF3ucGpBmAm6VaIO0upjysbKL+g05WRj5BKsHp2a2DfMlzp+TcDbpMcy/4YXYwA+BGIilIKeFbR',
'AkWDT6MP/mLfh0ud4+xZpdymS1Qvq4AzasRVQatVWZpaVWOpGzjF5KJkzhWz4DHAnL5Q== m@e'
].join('')
});
}
}
References:
- CfnKeyPair (construct) in the CDK documentation.
- Amazon EC2 key pairs and Linux instances in the EC2 user guide.
Image credit: Kylemore Abbey, by Lode Van de Velde. In the Public Domain. Source.
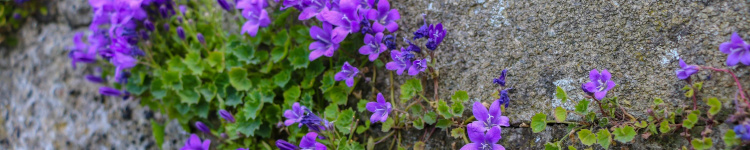
Migrations, tests and runserver in Django reusable apps
How do you create migration files, run unit tests and preview your Django app in the browser when developing a reusable app that is not nested inside a Django project?
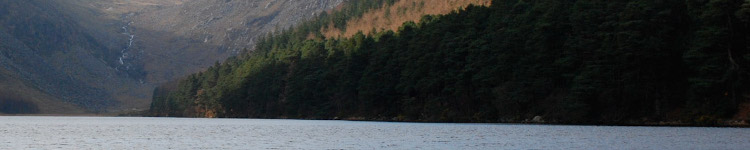
Validate that only one of many parameters was provided in Python
Sometimes, a function or class constructor can take many possible parameters, but only one parameter can have a value
at any one time, excluding all others. One way to validate this is included in the Python standard library. Copying
from the uuid.py
module:
class UUID:
def __init__(self, hex=None, bytes=None, bytes_le=None, fields=None,
int=None, version=None,
*, is_safe=SafeUUID.unknown):
if [hex, bytes, bytes_le, fields, int].count(None) != 4:
raise TypeError('one of the hex, bytes, bytes_le, fields, '
'or int arguments must be given')
The idea here is that since all five mutually exclusive parameters hex
, bytes
, bytes_le
, fields
and int
have a default value of None
, when one of them is specified during instatiation, there will then be four
parameters left with value None
.
Image credit: Wicklow Mountains, by Marcus Tan. In the Public Domain. Source.
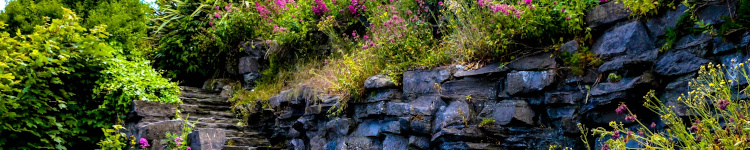
Apache host configuration for a Django website
This is the Apache virtual host configuration for an older project that I don't use any more.
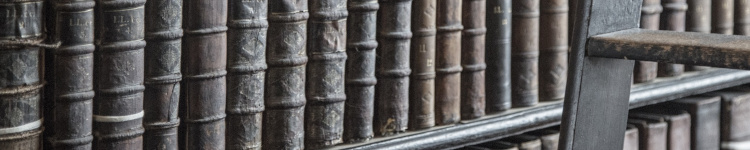
Mount an NTFS disk in Ubuntu
On a system that dual boots Windows and Ubuntu, I use this line in /etc/fstab
to have the NTFS Windows disk mounted
permanently in Ubuntu:
/dev/disk/by-uuid/1EBB57A121AFC448 /mnt/1TB auto nosuid,nodev,nofail,x-gvfs-show,uid=marios,gid=marios,permissions,dmask=022,fmask=133 0 0
Here's a list of options used in that line:
nosuid
: this is a security enhancement, preventing execution of files under the files' owner's permissios, from users other than the ownernodev
: this prevents the mounted filesystem from containing device filesnofail
: prevents error reports when the device is missing, but can mask failuresx-gvfs-show
: makes the device visible in the user interface, in the file exploreruid=marios
andgid=marios
: assign user and group ownership of new files to a specific userdmask=022
andfmask=133
: default permissions for newly created files and directories
Image credit: Library At Trinity College. In the Public Domain. Source.
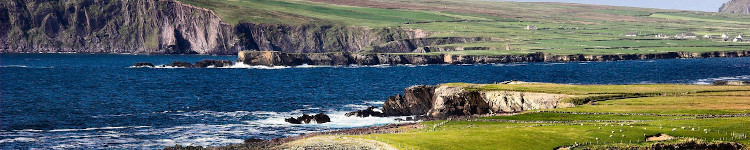
My .vimrc
This is my pretty basic ~/.virmc
. Lines starting with a double quotation mark are comments:
" Make text pop out on dark backgrounds:
set bg=dark
" Always display line numbers:
set number
" TAB width is 4:
set tabstop=4
" ...and so is an INDENT:
set shiftwidth=4
" Make TAB insert an INDENT at the beginning of a line:
set smarttab
" TAB is 4 SPACES instead of one \t:
set expandtab
set modeline
Image credit: Wild Grazing - Sheep grazing on the southwest coast of Ireland. In the Public Domain. Source.
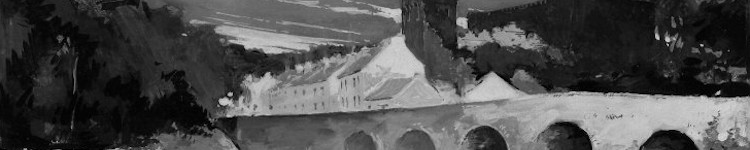
A small web application with Angular5 and Django
Django works well as the back-end of an application that uses Angular5 in the front-end. In my attempt to learn
Angular5 well enough to build a small proof-of-concept application, I couldn't find a simple working example of a
combination of the two frameworks, so I created one. I called this the Pizza Maker. It's available on GitHub, and
its documentation is in the README
.
If you have any feedback for this, please open an issue on GitHub.
Bootstrap
Dashboard Templates
This is a list of free (an in beer) Dashboard or Admin templates build on Bootstrap4. Some are released under well-known open source licenses (mostly MIT), and some have other restrictions, however all are gratis. These links tend to break over time, but often there are mirrors of the themes elsewhere, at least for the open source ones.
Angular
Getting Started with Typescript
A series of videos from codingforentrepreneurs.com on getting started with TypeScript.
- Welcome
- Install Node.js
- Configure TypeScript
- Classes - Part 1
- Classes - Part 2
- Getting Started with Typescript - Declaring Variables - let vs var
- Getting Started with Typescript - Run & Compile with Webpack
- Getting Started with Typescript - TypeScript - Classes - Part 3
- PackageJSON - npm init
- jQuery, Typings, & npm
- Thank you
Try Angular 4
A good series of videos from codingforentrepreneurs.com guiding through the creation of a complete application in Angular 4:
- Welcome (2:40)
- Getting Started (5:53)
- App Module & Component (8:13)
- Ng Generate New Component (5:46)
- Selectors & Components (2:08)
- ngFor and ngIf (7:57)
- Mapping Urls with RouterModule (9:17)
- Dynamic Routing of Components (7:38)
- Safely Embed a Video (8:38)
- Pipes & Custom Pipes (9:11)
- Rapid Bootstrap 3 Overview (15:14)
- Bootstrap for Angular // ngx bootstrap (11:39)
- ngx bootstrap carousel (11:56)
- Angular Click Events (14:17)
- Http Requests (17:02)
- Http & Featured (6:22)
- Two Way Data Binding (4:22)
- ngForm Basics (7:55)
- Search Detail (12:09)
- Passing Data to Components (12:08)
- Video Service (16:47)
- Search Video List (8:01)
- Video Item Model (7:19)
- Router Link & Improved Navigation (4:16)
- Improve Styling (11:06)
- ngClass (6:49)
- ngBuild and Deploy to Heroku (22:16)
- Thank You and Next Steps (1:43)
These instructions should get this up and running on Ubuntu:
git clone https://github.com/codingforentrepreneurs/Try-Angular-v4.git
cd Try-Angular-v4
npm install
ng serve